Balance de ruche à 30€ : épisode 4 - Le programme
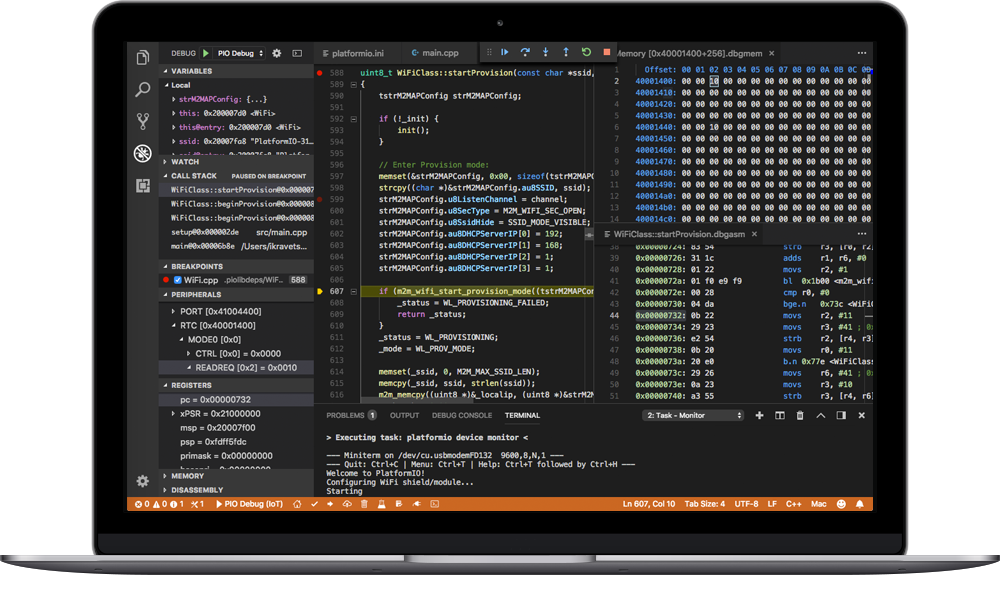
Je colle le code ici en mode sans explication. À savoir qu'il faudra adapter le BAL_CAL_FACTOR et le BAL_OFFSET à votre capteur .. partie qui devra être détaillée dans l'épisode 3 et forcément renseigner la partie OTAA avec les infos LoRaWAN.
Si vous voulez contribuer à ces articles, ce qui aidera beaucoup : cyrille.colin@educ.cloud
#include "LoRaWan_APP.h"
#include "Arduino.h"
#include "string.h"
#include <CayenneLPP.h>
#include <OneWire.h>
#include <DallasTemperature.h>
#include <HX711_ADC.h>
//HX711 constructor (dout pin, sck pin):
HX711_ADC LoadCell(GPIO1, GPIO2);
#define ONE_WIRE_BUS GPIO5
OneWire oneWire(ONE_WIRE_BUS);
DallasTemperature sensors(&oneWire);
float BAL_CAL_FACTOR = 23000.0;
long BAL_OFFSET = 8522000;
/* OTAA para*/
uint8_t devEui[] = {};
uint8_t appEui[] = {};
uint8_t appKey[] = {};
/* ABP para*/
uint8_t nwkSKey[] = {};
uint8_t appSKey[] = {};
uint32_t devAddr = (uint32_t)0x000000000;
/*LoraWan channelsmask*/
uint16_t userChannelsMask[6] = {0x00FF, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000};
/*LoraWan region, select in arduino IDE tools*/
LoRaMacRegion_t loraWanRegion = LORAMAC_REGION_EU868;
/*LoraWan Class, Class A and Class C are supported*/
DeviceClass_t loraWanClass = CLASS_A;
/*the application data transmission duty cycle. value in [ms].*/
uint32_t appTxDutyCycle = 10 * 60 * 1000; // 10 minutes
/*OTAA or ABP*/
bool overTheAirActivation = true;
/*ADR enable*/
bool loraWanAdr = true;
/* set LORAWAN_Net_Reserve ON, the node could save the network info to flash, when node reset not need to join again */
bool keepNet = true;
/* Indicates if the node is sending confirmed or unconfirmed messages */
bool isTxConfirmed = false;
/* Application port */
uint8_t appPort = 2;
uint8_t confirmedNbTrials = 4;
CayenneLPP lpp(160);
/* Prepares the payload of the frame */
static void prepareTxFrame(uint8_t port)
{
// Vext ON
digitalWrite(Vext, LOW);
sensors.requestTemperatures();
LoadCell.update();
float voltage = getBatteryVoltage();
float weight = LoadCell.getData();
Serial.print("Battery voltage : ");
Serial.println(voltage);
Serial.print("Weight : ");
Serial.println(weight);
lpp.reset();
lpp.addAnalogInput(1, voltage / 1000);
lpp.addAnalogInput(2, weight);
lpp.addTemperature(3, sensors.getTempCByIndex(0));
appDataSize = lpp.getSize();
memcpy(appData, lpp.getBuffer(), lpp.getSize());
// Vext OFF
digitalWrite(Vext, HIGH);
}
void setup()
{
boardInitMcu();
Serial.begin(115200);
pinMode(Vext, OUTPUT);
deviceState = DEVICE_STATE_INIT;
LoRaWAN.ifskipjoin();
// Vext ON
digitalWrite(Vext, LOW);
// Start up the library
sensors.begin();
LoadCell.begin();
LoadCell.start(2000, false);
LoadCell.setTareOffset(BAL_OFFSET);
LoadCell.setCalFactor(BAL_CAL_FACTOR);
while (!LoadCell.update());
}
void loop()
{
switch (deviceState)
{
case DEVICE_STATE_INIT:
{
printDevParam();
LoRaWAN.init(loraWanClass, loraWanRegion);
deviceState = DEVICE_STATE_JOIN;
break;
}
case DEVICE_STATE_JOIN:
{
// LoRaWAN.displayJoining();
LoRaWAN.join();
break;
}
case DEVICE_STATE_SEND:
{
// LoRaWAN.displaySending();
prepareTxFrame(appPort);
LoRaWAN.send();
deviceState = DEVICE_STATE_CYCLE;
break;
}
case DEVICE_STATE_CYCLE:
{
// Schedule next packet transmission
txDutyCycleTime = appTxDutyCycle + randr(0, APP_TX_DUTYCYCLE_RND);
LoRaWAN.cycle(txDutyCycleTime);
deviceState = DEVICE_STATE_SLEEP;
break;
}
case DEVICE_STATE_SLEEP:
{
// LoRaWAN.displayAck();
LoRaWAN.sleep();
break;
}
default:
{
deviceState = DEVICE_STATE_INIT;
break;
}
}
}
PS : Si vous avez aimé cet article (et que vous êtes arrivés jusqu’ici), vous aimerez peut-être mon livre… Bon, ça n’a strictement rien à voir, mais il est franchement cool. Et si le cœur vous en dit, vous pouvez même l’acheter ici. Merci pour le soutien !
Amazon.fr - BULLSHIT, Manuel de Survie: Anatomie d’un monde qui tourne à vide - Colin, Cyrille - Livres
Noté /5. Retrouvez BULLSHIT, Manuel de Survie: Anatomie d’un monde qui tourne à vide et des millions de livres en stock sur Amazon.fr. Achetez neuf ou d’occasion